Beginner Level
Intermediate Level
Advanced Level
Introduction
JSON, which stands for JavaScript Object Notation, is a widely used data format that is easily readable by both humans and machines. With Python's built-in support for JSON, you can easily manipulate and work with JSON data in your scripts and programs. This tutorial will provide you with a comprehensive overview of working with JSON in Python, covering everything from importing and exporting JSON data to parsing JSON objects and accessing their individual elements.
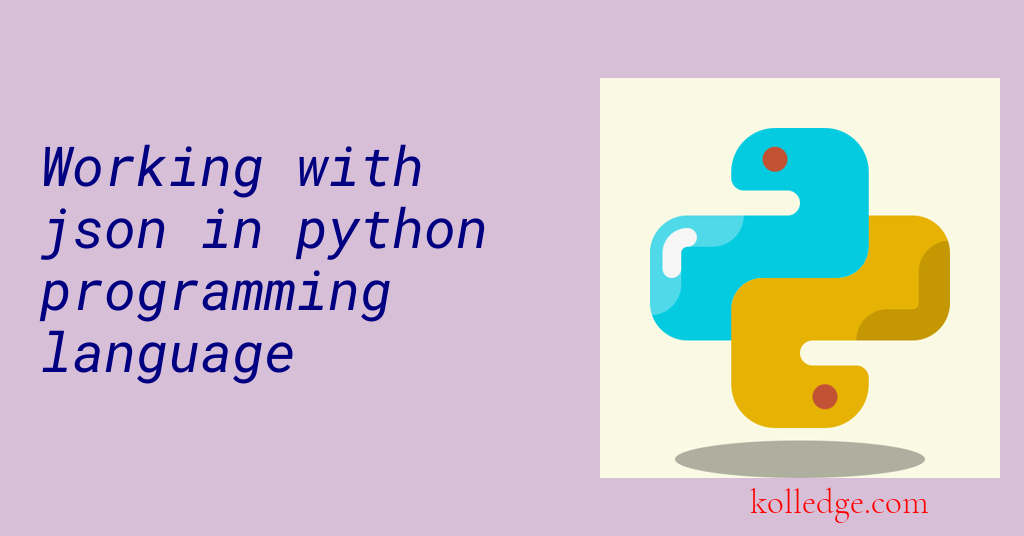
Table of Contents :
- Encoding JSON Data in Python
- Decoding JSON Data in Python
- Reading JSON Data from a File
- Writing JSON Data to a File
- Handling Errors in JSON
Encoding JSON Data in Python :
- Python has a built-in module called
json
for working with JSON data. - To encode Python objects to JSON, use the
json.dumps()
method. - Code Sample :
import json
person = {
"name": "John",
"age": 30,
"isStudent": True,
"subjects": ["English", "Maths", "Science"],
"address": {
"street": "123 Main St",
"city": "New York",
"state": "NY",
"zip": "10001"
}
}
json_data = json.dumps(person)
print(json_data)
Decoding JSON Data in Python :
- To decode JSON data to Python objects, use the
json.loads()
method. - Code Sample :
import json
json_data = '{"name": "John", "age": 30, "isStudent": true, "subjects": ["English", "Maths", "Science"], "address": {"street": "123 Main St", "city": "New York", "state": "NY", "zip": "10001"}}'
person = json.loads(json_data)
print(person)
Reading JSON Data from a File :
- To read JSON data from a file, use the
json.load()
method. - Code Sample :
import json
with open('person.json', 'r') as f:
person = json.load(f)
print(person)
Writing JSON Data to a File :
- To write JSON data to a file, use the
json.dumps()
method. - Code Sample :
import json
person = {
"name": "John",
"age": 30,
"isStudent": True,
"subjects": ["English", "Maths", "Science"],
"address": {
"street": "123 Main St",
"city": "New York",
"state": "NY",
"zip": "10001"
}
}
with open('person.json', 'w') as f:
json.dump(person, f)
Handling Errors in JSON :
- When working with JSON data, errors can occur when encoding or decoding data.
- The
json
module provides two exceptions:JSONDecodeError
andJSONEncodeError
. - These exceptions can be caught and handled to notify users of any issues.
- Code Sample :
import json
json_data = 'invalid json data'
try:
person = json.loads(json_data)
except json.JSONDecodeError as e:
print(f"Error decoding JSON data: {e}")
Prev. Tutorial : What is json
Next Tutorial : Reading json file