Introduction
Python provides a powerful feature called *args, which allows you to create functions that can accept a variable number of arguments. With *args, you can pass any number of arguments to a function, providing flexibility and convenience in your code. Whether you need to work with a fixed number of arguments or handle unpredictable input, *args is a valuable tool in your Python programming arsenal. In this tutorial, we will explore how to define and use variadic functions using *args, and discover practical examples where this feature can simplify your code and make it more adaptable. Let's dive in and master the art of variadic functions in Python!
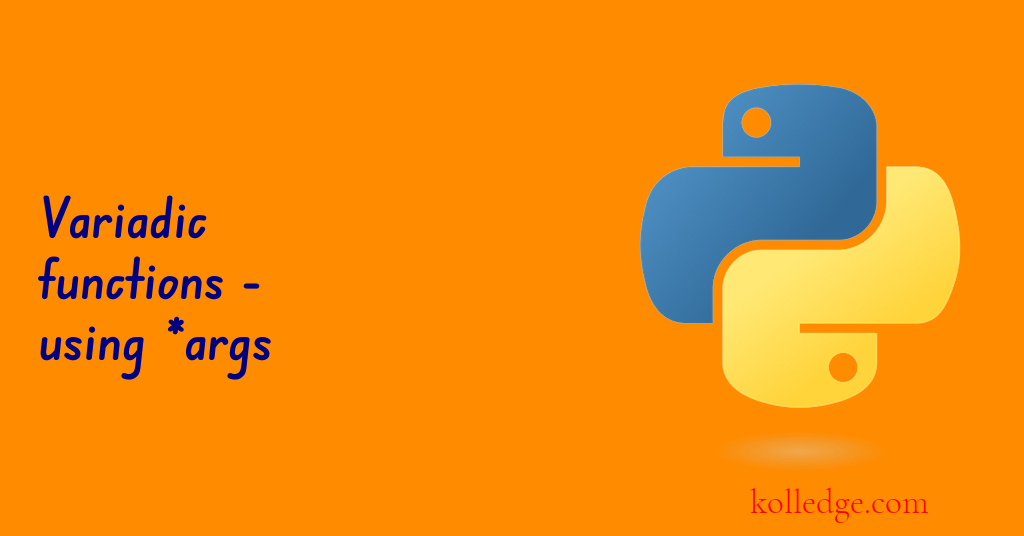
Table of Contents :
- Using *args in Python
- Variadic Parameter - *args
Using *args in Python :
- We have read about tuple unpacking in the previous tutorial.
- In case of the following tuple unpacking statement -
a, b, *c = 1, 2, 3, 4, 5
- 1 is assigned to a
- 2 is assigned to b
- [3, 4, 5] is assigned to c
- This same concept is similarly applicable to function arguments as well.
- Suppose we have a function definition :
def my_func(a, b, *c):
# function body
- And we make a call to the function using positional arguments like this :
my_func(1, 2, 3, 4, 5)
- In this case :
- 1 is assigned to a
- 2 is assigned to b
- Tuple (3, 4, 5) is assigned to c
- The only difference in case of function call is that in case of function arguments the args argument is a tuple and not a list.
- Code Sample :
def my_func(a, b, *c):
print("a = ", a)
print("b = ", b)
print("c = ", c)
my_func(10, 20, 30, 40, 50)
# Output
# a = 10
# b = 20
# c = (30, 40, 50)
Variadic Parameter - *args :
- The special parameter *args of a function is called a variadic parameter.
- It is a convention to name the variadic parameter as *args but it is not mandatory to name it as args.
- We can name it as anything e.g. *names, *ids, *price etc.
- Functions that have variadic parameter in their definition are called variadic functions.
- Variadic functions can take variable number of arguments - i.e. zero or more arguments.
- To access the values of the *args argument we use the square bracket notation :
- args[0]
- args[1]
- args[2]
- Also we can use the for loop to iterate over elements of variadic parameter.
- We cannot have any positional argument after variadic parameter.
- Only keyword arguments are allowed after variadic arguments.
- Code Sample :
def my_func(a, b, *args):
print("Value of a = ", a)
print("Value of b = ", b)
print("Value of arg_1 = ", args[0])
print("Value of arg_2 = ", args[1])
print("Value of arg_3 = ", args[2])
print()
print("Iterating over args")
# using for loop
for arg in args:
print(arg)
my_func(10, 20, 30, 40, 50)
# Output
# Value of a = 10
# Value of b = 20
# Value of arg_1 = 30
# Value of arg_2 = 40
# Value of arg_3 = 50
# Iterating over args
# 30
# 40
# 50
Prev. Tutorial : Unpacking Tuples
Next Tutorial : Keyword parameters