Beginner Level
Intermediate Level
Advanced Level
Introduction
One of the key features of SQL is the `ORDER BY` clause, which allows you to sort the results of a query based on one or more columns. In this tutorial, we will explore how to use the `ORDER BY` clause in Python to sort data stored in a SQL database using the `sqlite3` module in Python. We will cover the basics of connecting to a database, creating tables, inserting data, and performing queries with `ORDER BY` clause.
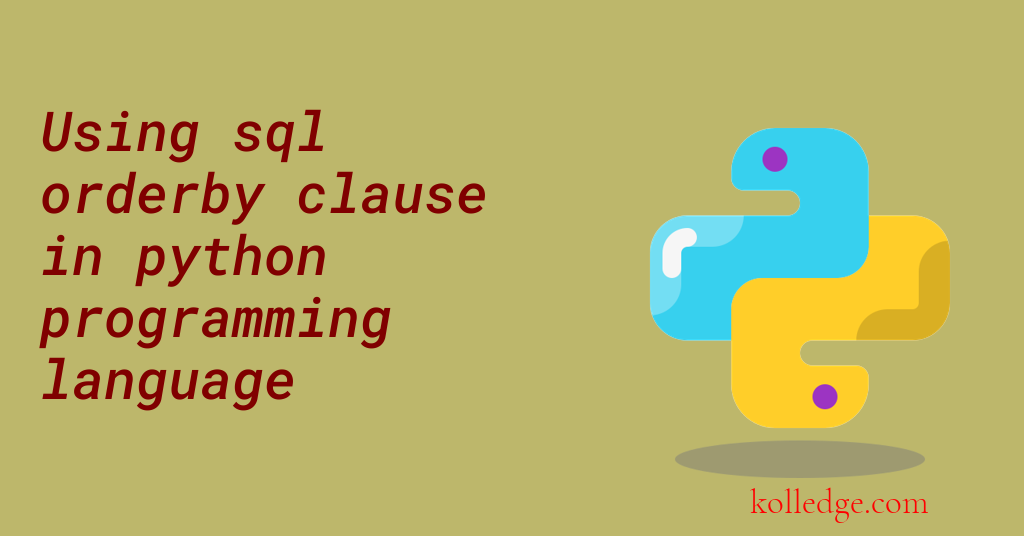
Table of Contents :
- What is Sql Orderby Clause
- How to use sql orderby clause in MySQL with Python
- Step 1: Install the required Packages
- Step 2: Establishing a Connection to the MySQL Server
- Step 3: Create a Cursor Object
- Step 4: use sql orderby clause
What is Sql Orderby Clause :
- The sql orderby clause allows you to sort the results of a query by one or more columns.
- The orderby clause is added to the end of a query.
- Orderby clause can be used to :
- sort the results in ascending order
- sort the results in descending order
- sort the results by multiple columns
- Ascending order : The following query sorts the results by the last_name column in ascending order
SELECT * FROM contacts ORDER BY last_name;
- Descending order : We can also sort the results in descending order by using the DESC keyword:
SELECT * FROM contacts ORDER BY last_name DESC;
- Multiple columns : We can sort the results by multiple columns by separating the column names with a comma:
SELECT * FROM contacts ORDER BY last_name, first_name;
How to use sql orderby clause in MySQL with Python :
- Follow the steps given below to use sql orderby clause in MySQL with python
- Step 1: Install the required Packages
- Step 2: Establishing a Connection to the MySQL Server
- Step 3: Create a Cursor Object
- Step 4: use sql orderby clause
Step 1: Install the Required Packages
- The first step is to ensure that you have installed the required packages on your machine.
- The most popular packages for connecting to MySQL in Python are
- mysql-connector and
- PyMySQL.
- You can install these packages using pip through the command line.
- Code Sample :
!pip install mysql-connector-python
!pip install PyMySQL
Step 2: Connect to the MySQL Database
- To create a new database in MySQL using Python, we must have a connection to the MySQL server.
- To connect to a MySQL server in Python we can use either
- mysql.connector or
- PyMySQL package
- We need to provide the necessary connection parameters to establish the connection.
- We need to pass the following parameters to the Connection object:
- host : the hostname of the MySQL server user
- user : username for the MySQL account
- password : password for the MySQL account database
- database : The name of the database to connect to
- To study package specific code for connecting to MySQL read our tutorial on connecting to MySQL server.
Step 3: Create a Cursor Object
- After establishing a connection to the MySQL server, your next step is to create a cursor object.
- This is done using the cursor() method provided by mysql.connector or PyMySQL.
- Code Sample :
cursor = mydb.cursor()
Step 4: Use sql orderby clause
- To sort the results of a
- Use the
- Use the
- Use the
- Code Sample :
query = "SELECT * FROM employees ORDER BY name ASC"
cursor.execute(query)
result = cursor.fetchall()
for row in result:
print(row)
Prev. Tutorial : Using sql where clause
Next Tutorial : Delete data from mysql