Introduction
While Python has the scope of variables defined within a function, using the global keyword ensures that the variable being accessed is from the global module, hence avoiding the creation of a local variable with the same name as the global variable. Using global keyword allows for code re-usability, readability, and maintainability. This tutorial will focus on how to use the global keyword in Python programming and its practical applications. By the end of the tutorial, you will have a solid understanding of global keyword and how to use them in your Python projects.
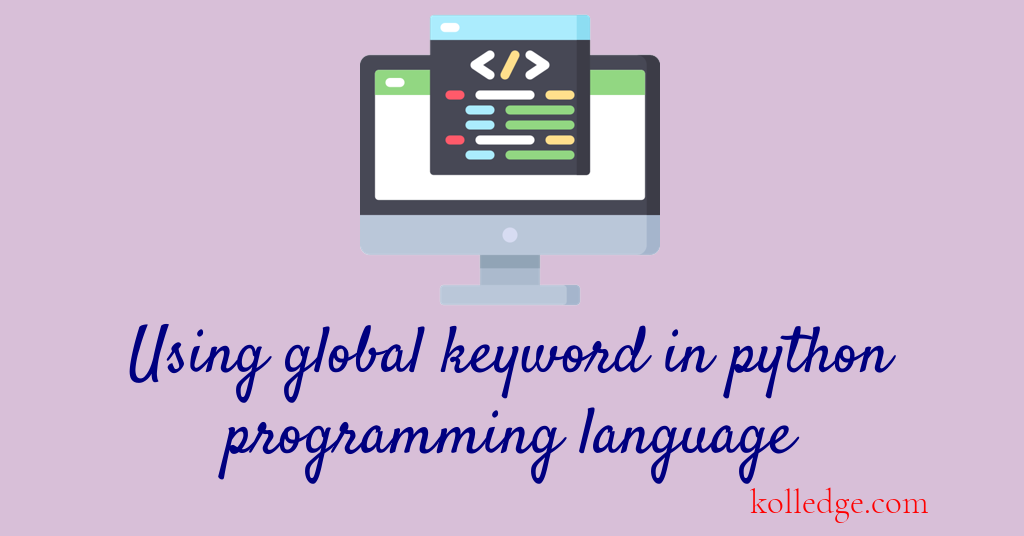
Table of Contents :
- Global Keyword
- Accessing a Global Variable
- Modifying Global variable
- Modifying global variable without using global keyword
- Modifying global variable using global keyword
Global Keyword
- We have already read about global and local variables in python.
- We know that global variables are variables that are declared outside of any function.
- Global variables can be accessed from anywhere in your code, inside or outside of functions.
- In Python, global variables are declared using the
global
keyword. - Note # : Note that we need to use the global keyword only if
- we want to modify the global variable.
- or we want to assign some value to the global variable.
- We do not need to use the global keyword for printing or accessing the global variables.
- Python interpreter treats any variable changed or created inside a function as a local variable unless it is explicitly declared as global.
Accessing a Global Variable :
- Here is a sample code for accessing global variables from a function :
- Note # :
- Note that we can easily access global variables from a function
- Using a global keyword is not necessary for accessing global variables.
- Sample Code :
glb = "I am Global."
def my_func():
print("Accessing global from inside : ", glb)
my_func()
# Output:
# Accessing global from inside : I am Global.
Modifying Global variable :
We can modify a global variable by two methods :
- without using global keyword
- using global keyword
Modifying global variable without using global keyword
- If you try to modify a global variable inside a function, without using the
global
keyword. - Note # :
- Note that value of glb has changed only inside
my_func
. - We have not used global keyword
- Hence
glb
is treated as a local variable withinmy_func
. - Outside the function the value of
glb
is still the same.
- Note that value of glb has changed only inside
- Sample Code :
glb = "I am Global"
def my_func():
glb = "I am local"
print("Accessing global from inside : ", glb)
my_func()
print("Accessing global from outside : ", glb)
# Output:
# Accessing global from inside : I am local
# Accessing global from outside : I am Global
Modifying global variable using global keyword
- If you try to modify a global variable inside a function using the
global
keyword. - Note # :
- Note that value of
glb
has changed outsidemy_func
as well. - We have used
global
keyword - Hence
glb
is treated as a global variable withinmy_func
as well.
- Note that value of
- Sample Code :
glb = "I am Global"
def my_func():
global glb
glb = "I am local"
print("Accessing global from inside : ", glb)
my_func()
print("Accessing global from outside : ", glb)
# Output:
# Accessing global from inside : I am local
# Accessing global from outside : I am local
Prev. Tutorial : Global and Local Variables
Next Tutorial : Nested functions