Beginner Level
Intermediate Level
Advanced Level
Introduction
As the demand for efficient and scalable applications grows, there is a need to develop robust programs that can handle multiple threads effectively. One of the ways to achieve this is through the use of a thread-safe queue. In this tutorial, we will explore the concept of thread-safe queues in Python and how they can be used to manage multiple threads concurrently while preventing data corruption and race conditions. We will examine the Python built-in queue module and demonstrate how to create, manipulate, and access thread-safe queues.
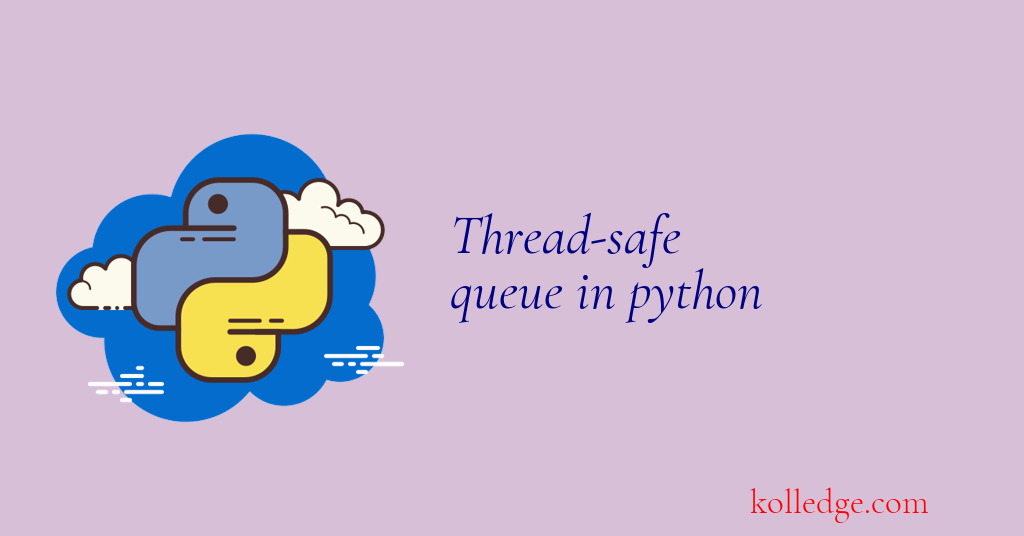
Table of Contents :
- Python Thread-Safe Queue
- Different Queue Operations
- Creating a New Queue in python
- Adding a new Item to the Queue
- Fetch an Item from the Queue
- Getting the Size of the Queue
- Marking a Task as Completed
- Waiting for All Tasks on the Queue to be Completed
- Implementing Python Thread-Safe Queue
Python Thread-Safe Queue :
- A thread-safe queue in Python is a data structure that can be used to communicate between multiple threads and ensure that data is being shared safely.
- The queue module in Python provides a Queue class that is thread-safe and can be used to implement a thread-safe queue.
Different Queue Operations :
- Different operations that we can perform on the queue are -
- Creating a New Queue in python
- Adding a new Item to the Queue
- Fetch an Item from the Queue
- Getting the Size of the Queue
Creating a New Queue in python
- To create a new queue, you can simply create a new instance of the
Queue
class from thequeue
module. - Code Sample :
from queue import Queue
# Create a new queue
q = Queue()
Adding a new Item to the Queue :
- To add an item to the queue, use the
put()
method. - Code Sample :
# Add an item to the queue
q.put(item)
Fetch an Item from the Queue :
- To get an item from the queue, use the
get()
method. - This method will block until an item is available in the queue.
- Code Sample :
# Get an item from the queue
item = q.get()
Getting the Size of the Queue :
- To get the size of the queue, use the
qsize()
method. - Code Sample :
# Get the size of the queue
size = q.qsize()
Marking a Task as Completed :
- To mark a task as completed, use the
task_done()
method. - Code Sample :
# Mark a task as completed
q.task_done()
Waiting for All Tasks on the Queue to be Completed :
- To wait for all tasks on the queue to be completed, use the
join()
method. - Code Sample :
# Wait for all tasks on the queue to be completed
q.join()
Implementing Python Thread-Safe Queue :
- Here, we create a thread-safe queue q and 5 worker threads using the worker() function as their target.
- We add
10
items to the queue using put(), then wait for all tasks to be completed usingjoin()
. - We then stop the worker threads by adding None items to the queue and waiting for the threads to finish using
join()
. - Code Sample :
import threading
import queue
def worker(q):
while True:
item = q.get()
if item is None:
break
print("Processing item", item)
q.task_done()
# Create a queue and worker threads
q = queue.Queue()
threads = []
for i in range(5):
t = threading.Thread(target=worker, args=(q,))
t.start()
threads.append(t)
# Add items to the queue
for item in range(10):
q.put(item)
# Wait for all tasks on the queue to be completed
q.join()
# Stop worker threads
for i in range(5):
q.put(None)
for t in threads:
t.join()
Prev. Tutorial : Daemon threads
Next Tutorial : Thread Pools