Beginner Level
Intermediate Level
Advanced Level
Introduction
Inheritance allows developers to create new classes based on existing ones, thereby reducing code duplication and improving code maintainability. In Python, developers can implement Multilevel Inheritance, which is a type of inheritance where a derived class is created from an existing derived class. This tutorial will explore the concept of Multilevel Inheritance in Python and provide a step-by-step guide on how to implement it in your programs.
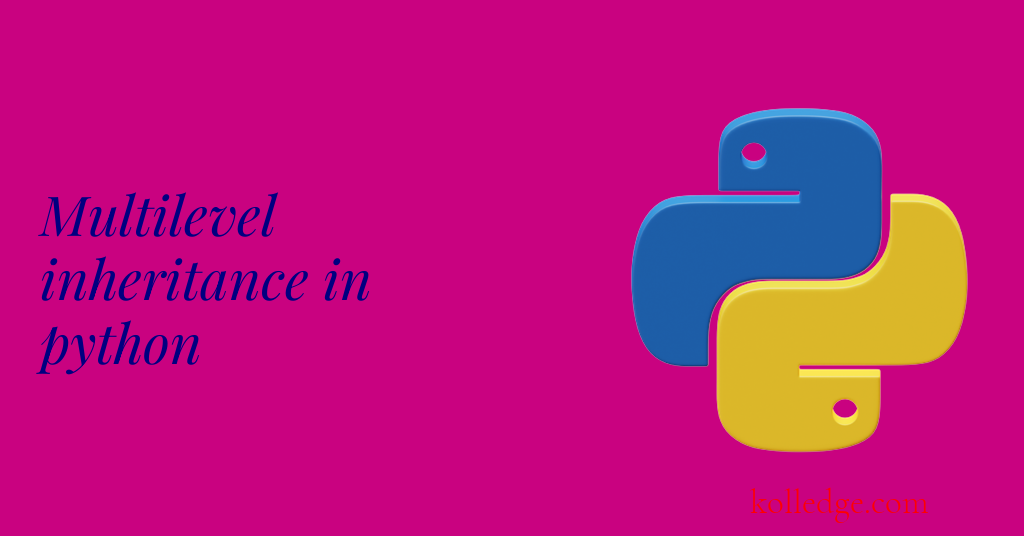
Table of Contents :
- What is Multilevel inheritance
- Creating a Subclass with Multilevel Inheritance
- Method Resolution Order (MRO)
- Diamond Inheritance
What is Multilevel inheritance :
- Multilevel inheritance is a type of inheritance where a sub-class is derived from another sub-class.
- It is a way of creating a hierarchy of classes in which each class inherits properties and methods from its parent class.
- In Python, we achieve multilevel inheritance using the `class` keyword and passing the superclass and parent class inside parentheses after the class name.
Creating a Subclass with Multilevel Inheritance :
- To create a subclass with multilevel inheritance, we define a new class and specify the parent class and superclass from which it will inherit using the `super()` function.
- Example:
class Animal:
def __init__(self, species):
self.species = species
def speak(self):
print("This animal speaks")
class Dog(Animal):
def __init__(self, name, breed):
self.name = name
self.breed = breed
super().__init__("Dog")
def speak(self):
print("This dog barks")
class Bulldog(Dog):
def __init__(self, name):
super().__init__(name, "Bulldog")
def speak(self):
super().speak()
print("This Bulldog growls")
b = Bulldog("Buddy")
b.speak()
# Output: This dog barks \n This Bulldog growls
Method Resolution Order (MRO) :
- In multilevel inheritance, Python determines the order in which the methods of the superclasses are called using MRO algorithm.
- The MRO algorithm follows a Depth First Search (DFS) approach to traverse the hierarchy of classes.
- We can view the MRO for a class using the `mro()` method.
- Example:
class A:
def speak(self):
print("This is A speaking")
class B(A):
pass
class C(B):
pass
print(C.mro())
# Output: [, , , ]
Diamond Inheritance :
- Diamond inheritance is a scenario where a sub-class inherits from two super-classes that have a common parent class.
- It can create ambiguity in method resolution order as Python tries to avoid calling the common parent class method twice.
- This ambiguity is resolved using the C3 linearization algorithm which is built into Python.
- Code Sample :
class A:
def speak(self):
print("This is A speaking")
class B(A):
pass
class C(A):
def speak(self):
print("This is C speaking")
class D(B, C):
pass
d = D()
d.speak()
# Output:
# This is C speaking
Prev. Tutorial : Multiple inheritance in Python
Next Tutorial : Hierarchical inheritance