Beginner Level
Intermediate Level
Advanced Level
Introduction
Dictionaries are powerful data structures in Python that allow for efficient and fast lookups of key-value pairs. However, there may be times when you need to change or delete certain entries in a dictionary. Whether you're a beginner or an experienced Python programmer, this tutorial will guide you through the different ways you can modify or delete dictionary entries in Python.
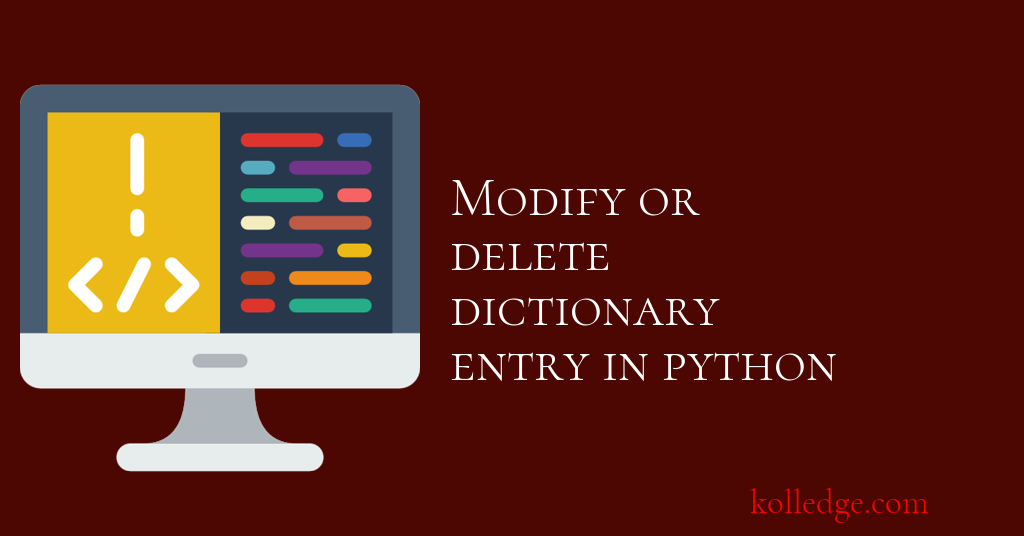
Table of Contents :
- Modifying the values of the key-value pairs
- Deleting a key-value pair
Modifying the values of the key-value pairs
- The basic syntax of modifying value of a key-value pair of the dictionary is :
d[key] = new_value
- Code Sample :
quantities = {
"apple": 10,
"oranges": 20,
"banana": 25,
"Mangoes": 35
}
print("Value os dictionary :")
print(quantities)
print()
quantities["apple"] = 5
print("Value os dictionary :")
print(quantities)
# Output
# Value os dictionary :
# {'apple': 10, 'oranges': 20, 'banana': 25, 'Mangoes': 35}
# Value os dictionary :
# {'apple': 5, 'oranges': 20, 'banana': 25, 'Mangoes': 35}
Deleting a key-value pair
- We can use the
del
keyword to delete an entry of the dictionary. - The basic syntax of deleting a key-value pair of the dictionary is :
del d[key]
quantities = {
"apple": 10,
"oranges": 20,
"banana": 25,
"Mangoes": 35
}
print("Value os dictionary :")
print(quantities)
print()
del quantities["apple"]
print("Value os dictionary :")
print(quantities)
# Output
# Value os dictionary :
# {'apple': 10, 'oranges': 20, 'banana': 25, 'Mangoes': 35}
# Value os dictionary :
# {'oranges': 20, 'banana': 25, 'Mangoes': 35}
Prev. Tutorial : Adding entry to a dictionary
Next Tutorial : Looping through a dict