Introduction
Python is a powerful and widely used programming language that offers a range of features, one of which is the ability to work with dictionaries. In Python, dictionaries are extremely useful for storing data that is structured as key-value pairs. However, accessing each element of a dictionary can sometimes be tricky, especially when the dictionary is large or complex. This is where looping through the elements of a dictionary comes in. By using a loop, you can easily traverse and manipulate all elements of a dictionary, regardless of its size or complexity. In this Python tutorial, we will learn how to loop through the elements of a dictionary using various methods and techniques.
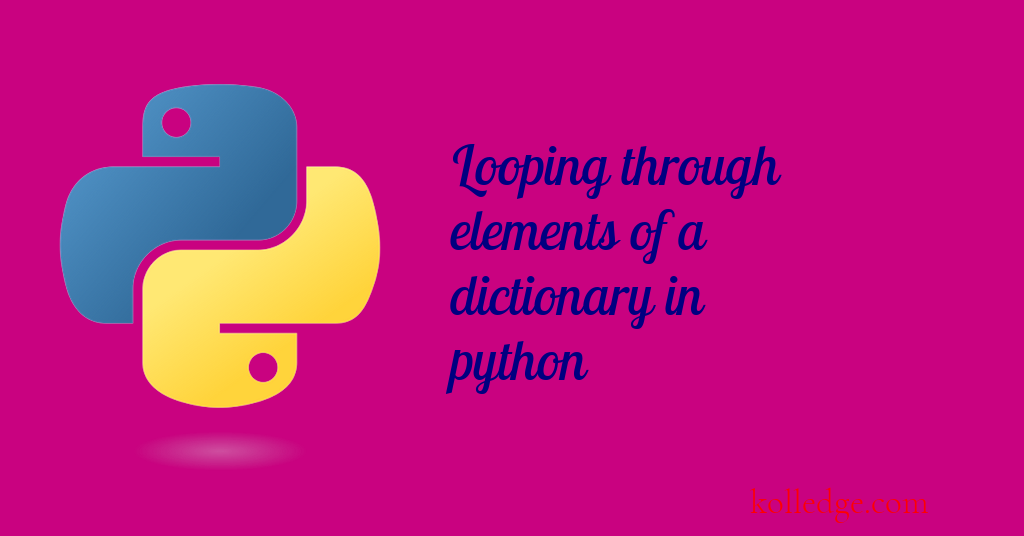
Table of Contents :
- Looping through all elements of a dictionary
- Looping through all keys of a dictionary
- Looping through all values of a dictionary
Looping through all elements of a dictionary :
- To loop through all key-value pairs of a dictionary we can use the
items()
method. - The
items()
method returns an object that contains a list of all key-value pairs of the dictionary. - The key-value pairs are returned as tuples by the
items()
method. - We can use a for loop with two variables to iterate over this list of key value pairs -
- The basic syntax of iterating items of dictionary is as follows :
for key, val in d.items():
# process key, val
- The name of the variables an be anything - not necessarily key and val.
- Code Sample :
quantities = {
"apple": 10,
"oranges": 20,
"banana": 25,
"Mangoes": 35
}
print("Looping through items of dictionary ")
for key, value in quantities.items():
print(f"Quantity of {key} = {value}",)
# Output
# Looping through items of dictionary
# Quantity of apple = 10
# Quantity of oranges = 20
# Quantity of banana = 25
# Quantity of Mangoes = 35
Looping through all keys of a dictionary :
- To loop through all keys of a dictionary we can use the
keys()
method. - The
keys()
method returns an object containing a list of all keys of the dictionary. - We can use a for loop to iterate over this list of keys.
- The basic syntax of iterating keys of dictionary is as follows :
for key in d.keys():
# process key
- Note # : iterating through all keys is the default iteration behavior of dictionary. Hence we can iterate over the dictionary directly.
- The
keys()
method comes in handy when we need a list of keys for some reason. - Code Sample :
quantities = {
"apple": 10,
"oranges": 20,
"banana": 25,
"Mangoes": 35
}
print("Looping through items of dictionary ")
for key in quantities.keys():
print(f"Quantity of {key} = {quantities[key]}")
print()
available_fruits = list(quantities.keys())
print("List of available fruits : ", available_fruits)
# Output
# Looping through items of dictionary
# Quantity of apple = 10
# Quantity of oranges = 20
# Quantity of banana = 25
# Quantity of Mangoes = 35
# List of available fruits : ['apple', 'oranges', 'banana', 'Mangoes']
Looping through all values of a dictionary :
- To loop through all values of a dictionary we can use the
values()
method. - The
values()
method returns an object containing a list of all values of the dictionary. - We can use a for loop or a while loop to iterate over this list of values.
- The basic syntax of iterating values of a dictionary is as follows :
for value in d.values():
# process value
- Code Sample :
quantities = {
"apple": 10,
"oranges": 20,
"banana": 25,
"Mangoes": 35
}
stock = 0
print("Calculating total stock")
for value in quantities.values():
stock = stock + value
print(f"Total fruits available = {stock}")
# Output
# Calculating total stock
# Total fruits available = 90
Prev. Tutorial : Modify/delete dict entry
Next Tutorial : Dictionary comprehension