Beginner Level
Intermediate Level
Advanced Level
Introduction
Iterators are essential for any programmer who wants to write efficient code that can handle large amounts of data. The Iter()
function is an in-built function in Python that returns an iterator for an object. This allows for more efficient and flexible iteration over data structures, making complex programming tasks much easier. In this tutorial, we will go over the basics of the Iter()
function and how it can be used to make your Python code more efficient and powerful.
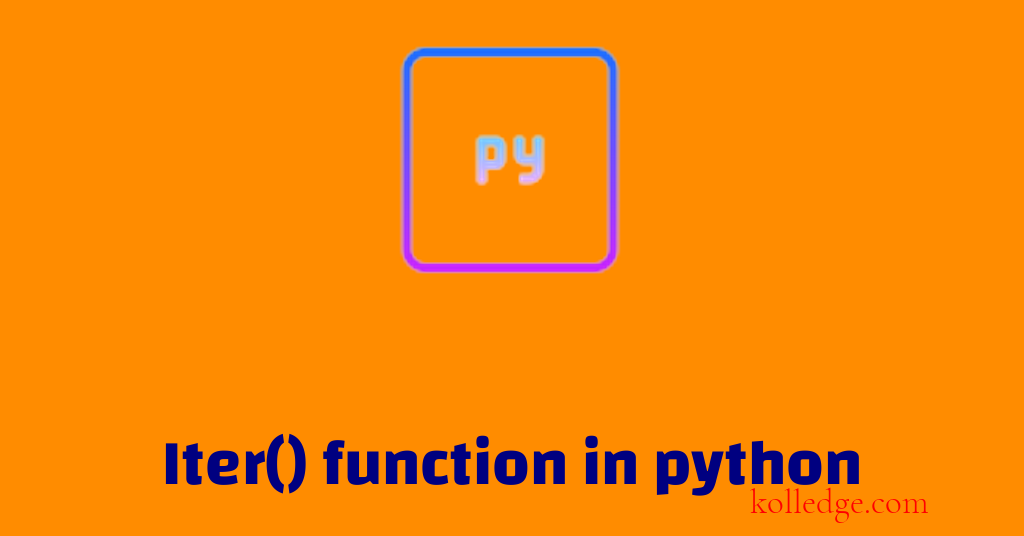
Table of Contents :
- Iter() function
- Code samples for Python iter() function
- The second form of the Python iter() function
- Test if an object is iterable
Iter() function :
- Python's
iter()
function is a built-in function in Python that returns an iterator for a given object. - It takes one or two arguments.
- The first argument is the object to be made into an iterator.
- The second argument is the sentinel, an optional value which when returned by the iterator, raises the
StopIteration
.
Code samples for Python iter() function :
- The simplest example of the
iter()
function creates an iterator from a list or tuple. - Code Sample :
my_list = [1, 2, 3, 4, 5]
my_iterator = iter(my_list)
# The next() method is used to get the next element from the iterator.
print(next(my_iterator)) Output: 1
print(next(my_iterator)) Output: 2
The second form of the Python iter() function :
- The second form of
iter()
function takes a callable function and sentinel. - The callable function is repeatedly called until it returns the sentinel.
- Once sentinel is returned,
StopIteration
is raised. - Code Sample :
def compare_values():
x = 5
while True:
y = yield
if y == x:
return True
g = compare_values()
next(g)
result = iter(g.send, 5) Stop when the generator returns 5
Test if an object is iterable :
- You can use Python's
iter()
function to test if an object is iterable. - When you call
iter()
on an object, Python will look for an__iter__()
method on the object. - If it's found, the object is considered iterable, otherwise, a
TypeError
is raised. - Code Sample :
def is_iterable(obj):
try:
iter(obj)
return True
except TypeError:
return False
print(is_iterable('Hello')) Output: True
print(is_iterable([1, 2, 3])) Output: True
print(is_iterable({'a': 1, 'b': 2})) Output: True
print(is_iterable(20)) Output: False
Prev. Tutorial : Iterators vs. Iterables
Next Tutorial : Custom Sequence Type