Introduction
Python is a programming language known for its versatility and ease of use. One of the most frequently used capabilities of Python is working with time and dates. Being able to get the current time is particularly useful for data science, web scraping, game development and many other applications. In this tutorial, we will explore how to get the current time in the Python programming language. We will cover the different methods available for retrieving the current time, including the datetime and time modules in Python. By the end of this tutorial, you will have a solid grasp of how to retrieve the current time and how to use it in your Python programs.
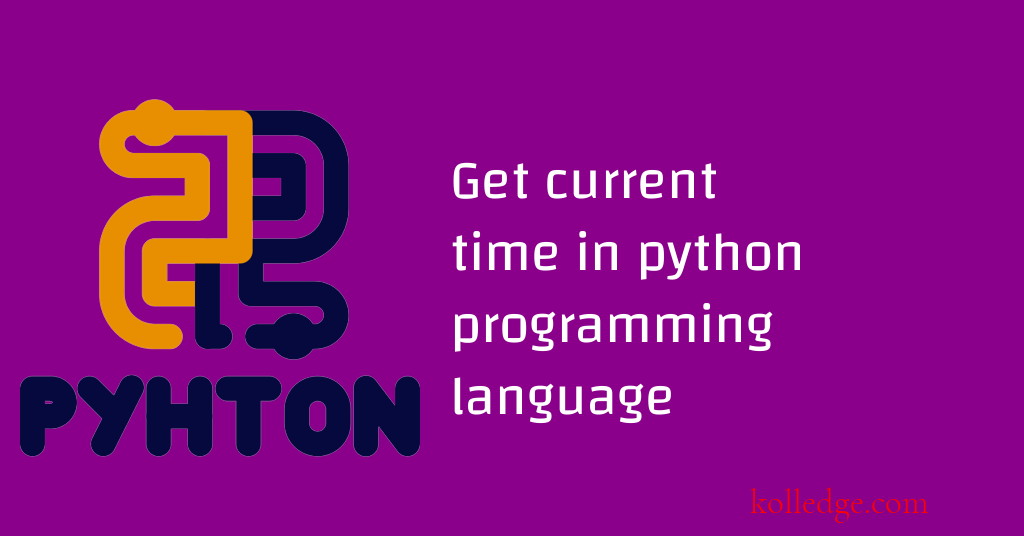
Table of Contents :
- Different ways of getting Current date and time in Python :
- Getting the current time using datetime object :
- get current time using now() function
- get current time in UTC using Datetime Object
- get current time from ISO format string
- Getting the current time using time Module :
- get current time using time() function
- get the current time as a string
- get current time in Milliseconds
- get current time in Nanoseconds
- get current time using localtime()
- get current GMT time
- get current time in Epoch
Different ways of getting current time in python :
- We can get the current time in python using the datetime module or the time module.
- Getting the current time using datetime object :
- get current time using now() function
- get current time in UTC using Datetime Object
- get current time from ISO format string
- Getting the current time using time Module :
- get current time using time() function
- get the current time as a string
- get current time in Milliseconds
- get current time in Nanoseconds
- get current time using localtime()
- get current GMT time
- get current time in Epoch
Get current time using datetime object
How to get current time using now() function
- The
datetime
class in Python's datetime module can be used to represent and manipulate dates and times together. - The
now()
method of the datetime class returns the current date and time as adatetime
object. - We can then use the
time()
function of this datetime object to get the current time. - Code Sample :
from datetime import datetime
now = datetime.now()
current_time = now.time()
print("Current time:", current_time)
# Output:
# Current time: 11:10:36.695786
How to get current time in UTC using datetime object
- The
pytz
module in python can be used to get date-time in different timezones. - We can then use the strftime() method to format dates and retrieve the time information.
- Code Sample : Below is the code to get the current time in UTC
from datetime import datetime
import pytz
utc_now = datetime.now(pytz.utc)
utc_time = utc_now.strftime('%H:%M:%S %Z')
print("UTC time :", utc_time)
# Output
# UTC time : 05:46:27 UTC
How to get current time from ISO format string
- suppose we have date-time in ISO format string,
- We can use the
fromisoformat()
method to convert this string to datetime object. - This datetime object can then be used to fetch the time information using the time() method.
- Code Sample :
from datetime import datetime
iso_datetime = "2021-07-14T11:37:30.172299"
curr_datetime = datetime.fromisoformat(iso_datetime)
curr_time = curr_datetime.time()
print("Current time in ISO format:", curr_time)
# Output:
# Current time in ISO format: 11:37:30.172299
Get current time using time module
- The time module in Python can also be used to get the current time.
- We can use import the time module using the statement :
import time
How to get the current time using time() function
- The
time
module'stime()
function can be used to get the current time. - the time() function returns current time as the number of seconds since the Unix Epoch.
- The date January 1st, 1970 at 00:00:00 UTC is referred to as the Unix epoch.
- Code Sample :
import time
current_time = time.time()
print("Current time using time() function :", current_time)
# Output
# Current time using time() function : 1689316431.7559302
How to get the current time as a string
- We can also use the ctime() function of the
time
module to get a string representation of the current time. - Code Sample :
import time
current_time = time.ctime()
print("Current time :", current_time)
# Output
# Current time : Fri Jan 12 12:05:40 2021
How to get current time in milliseconds using time:
- The
time()
function can be used with round() to get the current time in milliseconds. - Code Sample :
import time
current_time = round(time.time() * 1000)
print("Current time in milliseconds:", current_time)
# Output
# Current time in milliseconds: 1646151284570
How to get current timein nanoseconds using time:
- The
time()
function can also be used withperf_counter_ns()
from thetime
module to get the current time in nanoseconds. - Code Sample :
import time
current_time = time.perf_counter_ns()
print("Current time in nanoseconds:", current_time)
# Output
# Current time in nanoseconds: 15904500
How to get the current time using localtime() function :
- The localtime() function of the
time
module can be used to get the current time. - The
localtime()
function returns time instruct_time
format. - We can use this format to get the current time.
- The process of obtaining current time from
localtime()
function is -- use the
localtime()
function to get time instruct_time
format. - convert this
struct_time
format to to epoch time using the mktime() function. - convert this epoch time to
datetime
object using thefromtimestamp()
function. - from this
datetime
object extract the current time using thetime()
function.
- use the
- Code Sample :
import time
from time import mktime
from datetime import datetime
local_time = time.localtime()
epoch_time = mktime(local_time)
curr_datetime = datetime.fromtimestamp(epoch_time)
curr_time = curr_datetime.time()
print("Current time :", curr_time)
# Output
# Current time : 12:02:05
How to get current GMT time using time:
- The
gmtime()
function of thetime
module can be used to get the current GMT time. - The
gmtime()
function returns time instruct_time
format. - The process of obtaining current time from
gmtime()
function is same as we extracted current time from thelocaltime()
function in the previous section. - Code Sample :
import time
from time import mktime
from datetime import datetime
current_gmt = time.gmtime()
epoch_time = mktime(current_gmt)
curr_datetime = datetime.fromtimestamp(epoch_time)
curr_time = curr_datetime.time()
print("Current GMT time:", curr_time)
# Output
# Current GMT time: 06:45:35
How to get current Time In Epoch using Time:
- The
time()
function can also be used to get the current time in the Unix epoch format. - The epoch is the point where the time starts, and is usually expressed as the number of seconds since 1 January 1970 00:00:00 UTC.
- Code Sample :
import time
current_time = int(time.time())
print("Current time in epoch format:", current_time)
# Output
# Current time in epoch format: 1189317173
Prev. Tutorial : Current date-time
Next Tutorial : Timestamp