Beginner Level
Intermediate Level
Advanced Level
Introduction
As we start writing Python code, it's important to know how to display the results of your code in a clear and effective way. This tutorial will cover the different ways that Python allows you to output information to the console or to a file. From simple print statements to formatted strings, we will walk you through everything you need to know in order to display output in Python. So whether you are a beginner or an experienced Python developer, this tutorial will provide you with the knowledge you need to display Python output with confidence.
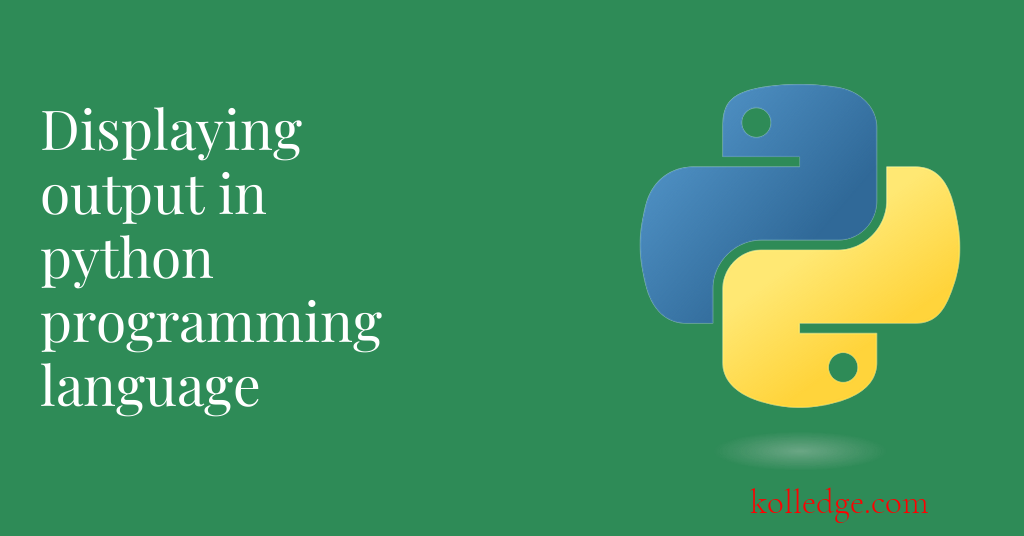
Table of Contents :
- Output in Python - print() function
- Displaying strings using print
- Displaying Integers and floats
- Displaying Lists using print
- Displaying Variables and Literals using print
Output in Python - print() function
- In Python we can display output using the print() function.
- We can display any type of data using the print() function e.g. strings, integers, floats, and lists etc.
Displaying strings using print
- To display strings pass the string enclosed in quotes to the print function.
- Here is a simple example of using the print() function to display a string:
print("Hello, world!")
# Output
# Hello, world!
- We can concatenate two strings within the print function before printing e.g.
print("Hello, " + "world!")
# Output
# Hello, world!
Displaying Integers and floats
- Integers and float numbers can also be displayed using the print() function as well :
print(42)
print(3.14159)
# Output
# 42
# 3.14159.
Displaying Lists using print
- Lists can be displayed using the print() function as follows :
my_list = [1, 2, 3, 4, 5]
print(my_list)
# Output
# [1, 2, 3, 4, 5].
Displaying Variables and Literals using print
- We can use print statement to display python variables and literals directly.
x = 10
print("The value of x = ", x)
print("I am a string literal")
# Output
# The value of x = 10
# I am a string literal
Prev. Tutorial : Taking input from user
Next Tutorial : Print statement