Beginner Level
Intermediate Level
Advanced Level
Introduction
Directories, also known as folders, are an essential part of file management and organization. Python's os module provides a wide range of functions to interact with directories. From creating, renaming, and deleting directories to retrieving the list of files and subdirectories in a directory, the os module offers a plethora of methods to handle directory operations. In this tutorial, we will walk through some of the most commonly used directory operations using the os module. By the end of this tutorial, you will have a solid understanding of how to perform directory operations in Python.
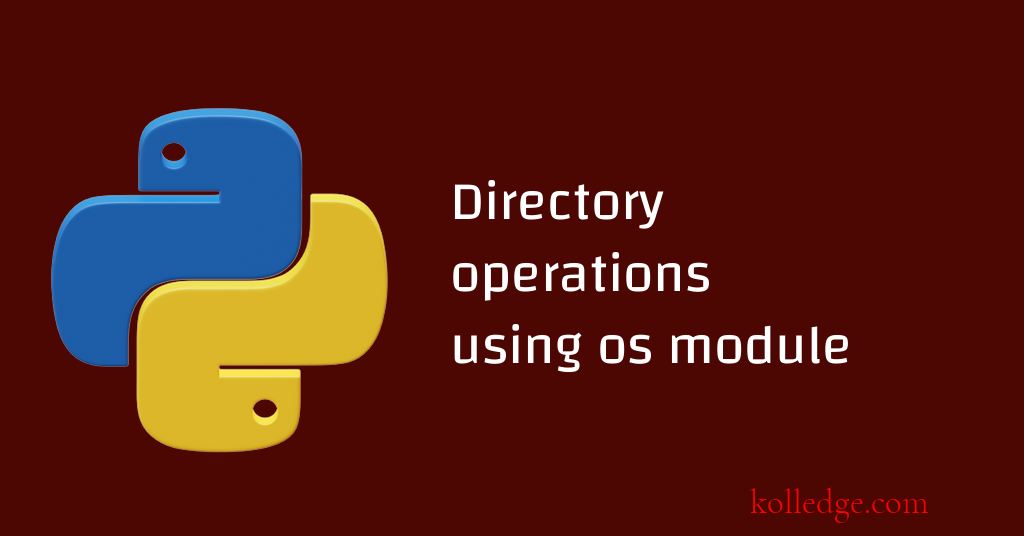
Table of Contents :
- How to create a directory in Python
- How to rename a Directory in Python
- How to delete an empty directory in Python
- How to delete a non-empty directory in Python
- How to traverse a directory recursively
How to create a directory in Python
- Use the
os.mkdir()
method to create a directory. - Code Sample :
import os
os.mkdir("new_directory")
How to rename a Directory in Python :
- Use the
os.rename()
method to rename a directory. - Code Sample :
import os
os.rename("old_directory", "new_directory")
How to delete an empty directory in Python :
- Use the
os.rmdir()
method to delete an empty directory. - Code Sample :
import os
os.rmdir("directory_to_be_deleted")
How to delete a non-empty directory in Python :
- Use the
shutil.rmtree()
method to delete a directory that contains files and/or sub-directories. - Code Sample :
import shutil
shutil.rmtree("directory_to_be_deleted")
How to traverse a directory recursively :
- Use the
os.walk()
method to traverse a directory recursively. - Code Sample :
import os
for root, dirs, files in os.walk("/path/to/directory"):
print("Directory:", root)
print("Sub-directories:", dirs)
print("Files:", files)
Prev. Tutorial : Path of a directory
Next Tutorial : List of files in a Dir.