Beginner Level
Intermediate Level
Advanced Level
Introduction
MySQL is an open-source relational database management system commonly used in web applications. Python is a powerful programming language known for its simplicity and ease of use. In this tutorial, we will be exploring how to establish a connection to a MySQL database using Python and how to create a table. This tutorial is suitable for beginners who are interested in learning how to use Python to interact with MySQL databases.
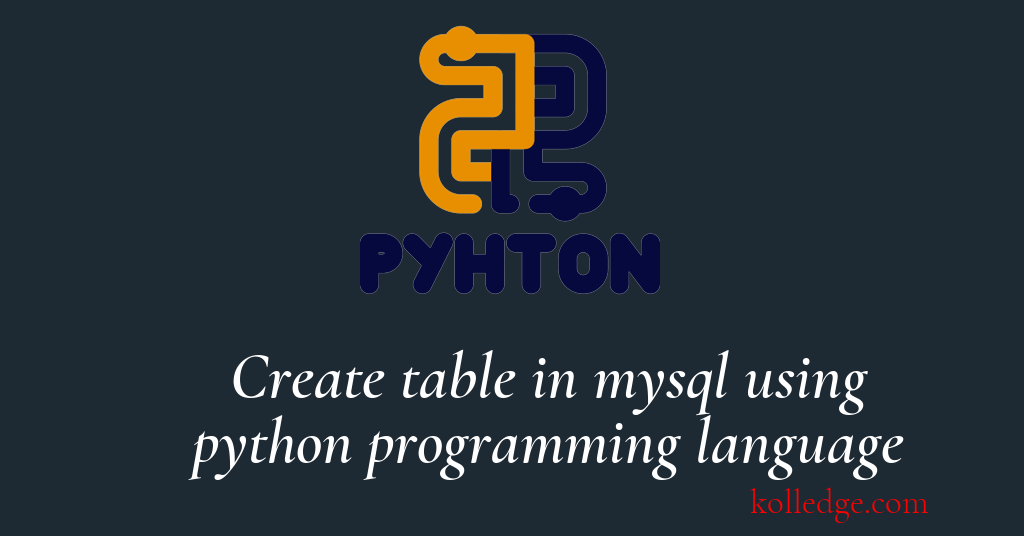
Table of Contents :
- How to create table in MySQL with Python
- Step 1: Install the required Packages
- Step 2: Establishing a Connection to the MySQL Server
- Step 3: Create a Cursor Object
- Step 4: Create a MySQL Table
- Step 5: Commit the changes
How to create table in MySQL with Python :
- Follow the steps given below to create table in MySQL with python
- Step 1: Install the required Packages
- Step 2: Establishing a Connection to the MySQL Server
- Step 3: Create a Cursor Object
- Step 4: Create a MySQL Table
- Step 5: Commit the changes
Step 1: Install the Required Packages
- The first step is to ensure that you have installed the required packages on your machine.
- The most popular packages for connecting to MySQL in Python are
- mysql-connector and
- PyMySQL.
- You can install these packages using pip through the command line.
- Example:
!pip install mysql-connector-python
!pip install PyMySQL
Step 2: Connect to the MySQL Database
- To create a new database in MySQL using Python, we must have a connection to the MySQL server.
- To connect to a MySQL server in Python we can use either
- mysql.connector or
- PyMySQL package
- We need to provide the necessary connection parameters to establish the connection.
- We need to pass the following parameters to the Connection object:
- host : the hostname of the MySQL server user
- username : username for the MySQL account
- password : password for the MySQL account database
- name of the database : Db name to connect to
- To study package specific code for connecting to MySQL read our tutorial on connecting to MySQL server.
Step 3: Create a Cursor Object
- After establishing a connection to the MySQL server, your next step is to create a cursor object.
- This is done using the cursor() method provided by mysql.connector or PyMySQL.
- Example:
cursor = mydb.cursor()
Step 4: Create a MySQL Table
- With a cursor object, you can now create a new table on the MySQL server.
- This is done using SQL query CREATE TABLE.
- Example:
# Create table
cursor.execute("CREATE TABLE customers (name VARCHAR(255), address VARCHAR(255))")
#Print message to confirm table creation
print("Table created successfully!")
Step 5: Commit the changes
- Finally, make sure to commit the changes to the MySQL table
- This can be done using the commit() method provided by mydb.
- Example:
mydb.commit()
#Print message to confirm changes
print("Changes successfully saved to the database!")
Prev. Tutorial : Create mysql database using Python
Next Tutorial : Drop table in mysql using Python