Beginner Level
Intermediate Level
Advanced Level
Introduction
Python, a versatile and powerful programming language, provides a rich set of arithmetic operators to perform various mathematical computations. From basic addition and subtraction to more complex operations like exponentiation and modulus, these operators allow you to solve a wide range of numerical problems efficiently. In this tutorial, we will explore the different arithmetic operators available in Python, understand their usage, and discover how they can be combined to perform complex mathematical calculations. So, let's dive in and unlock the potential of arithmetic operators in Python programming!
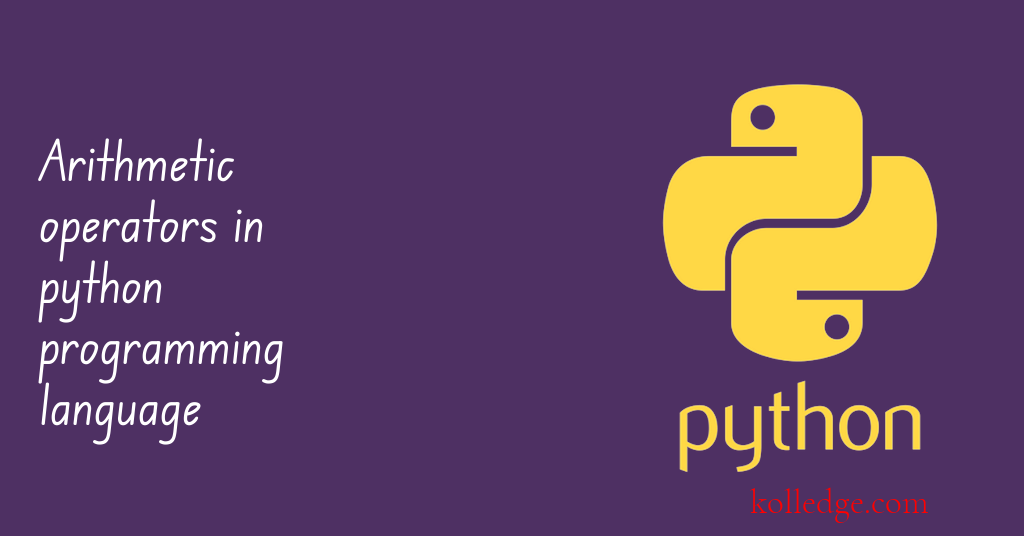
Table of Contents :
- Addition operator in Python (+)
- Using Addition operator for strings
- Subtraction operator in Python (-)
- Multiplication operator in Python (*)
- Using Multiplication operator for strings
- Division operator in Python (/)
- Floor division operator in Python (//)
- Modulus Operator in Python (%)
- Exponent Operator in Python (**)
- Arithmetic operators are used to perform mathematical operations on operands.
- Arithmetic operators in Python work in the same way as they do in mathematics.
- There are seven arithmetic operators in Python
- Addition Operator(+)
- Subtraction Operator(-)
- Multiplication Operator(*)
- Division Operator(/)
- Floor division Operator (//)
- Modulus Operator(%)
- Exponentiation Operator(**)
Addition operator in Python (+)
- Addition Operator is denoted by the plus sign '+'
- Addition operator adds two or more operands.
- It returns the sum of its operands as the result.
- Code Sample :
x = 10
y = 20
res = x + y
print(res)
# Output
# 30
# Here the addition operator adds x and y
# The sum is stored in the variable res
# The result 30 is then printed
Using Addition operator for strings in Python
- In Python the addition operator can also be used with strings.
- Addition operator concatenates its two or more string operands.
- Code Sample :
x = "Hello, "
y = "World!!!"
res = x + y
print(res)
# Output
# Hello, World!!!
# Here the addition operator concatenates strings x and y
# The sum is stored in the variable res
# Then prints the result
Subtraction operator in Python (-)
- Subtraction Operator is denoted by the minus sign '-'
- Subtraction operator is used to perform arithmetic subtraction operation between two operands.
- It subtracts the second operand from the first.
- Subtraction operator returns the difference between the values of operands as the result.
- Code Sample :
x = 20
y = 10
res = x - y
print(res)
# Output
# 10
# Here the Subtraction operator subtracts y from x
# The difference is stored in the variable res
# The result 10 is then printed
Multiplication operator in Python (*)
- Multiplication Operator is denoted by the asterisk sign '*'
- Multiplication operator multiplies two or more operands.
- It returns the product of its operands as the result.
- Code Sample :
x = 20
y = 10
res = x * y
print(res)
# Output
# 200
# Here the Multiplication operator multiplies x and y
# The product is stored in the variable res
# The result 200 is then printed
Using Multiplication operator for strings in Python
- In Python multiplication operator can also be used with strings.
- When used with strings multiplication operator simply repeats the string.
- Code Sample :
s = "str_"
n = 5
res = s * n
print(res)
# Output
# str_str_str_str_str_
# Here the Multiplication operator the string s
# The string s is repeated n number of times
# The result str_str_str_str_str_ is then printed
Division operator in Python (/)
- Division Operator is denoted by forward slash '/'.
- In a division operation
- the left operand is the dividend
- the right operand is the divisor
- Division operator divides the left operand by the right operand.
- Division Operator returns the quotient as the result.
- Division operator is used to perform floating-point arithmetic.
- Division Operator always returns a float value.
- The right operand of a division operator (divisor) can never be zero.
- If we'll use zero as divisor Python interpreter will give an error : 'ZeroDivisionError: division by zero'
- Code Sample :
x = 20
y = 10
res = x / y
print(res)
# Output
# 2.0
# Here the Division operator divides x by y
# The quotient is stored in the variable res
# The result 2.0 is then printed
Floor division operator in Python (//)
- Floor division operator is denoted by two forward slashes '//'.
- In a Floor division operation
- the left operand is the dividend
- the right operand is the divisor
- Floor division operator divides the left operand by the right operand.
- It returns the integer part of the quotient as the result.
- It rounds the result down to the nearest integer.
- This type of division is also called Integer division.
- Floor division operator is used to perform both floating-point and integer arithmetic.
- If both the operands are integers then it returns an integer value.
- If one of the operands is float then it returns float value.
- The right operand of a Floor division operator (divisor) can never be zero.
- If we'll use zero as divisor Python interpreter will give an error : 'ZeroDivisionError: integer division or modulo by zero'
- Code Sample :
x = 25
y = 10
res = x // y
print(res)
# Output
# 2
# Here the Division operator divides x by y
# The integer part of quotient is stored in the variable res
# The result 2 is then printed
Modulus Operator in Python (%)
- Modulus Operator is denoted by a percentage sign.
- In a Modulus operation
- the left operand is the dividend
- the right operand is the divisor
- Modulus operator divides the left operand by the right operand and returns the remainder as the result.
- Modulus operator can return both float or integer value depending on the operands.
- The right operand of a Modulus operator (divisor) can never be zero.
- If we'll use zero as divisor Python interpreter will give an error : 'ZeroDivisionError: integer division or modulo by zero'
- Code Sample :
x = 25
y = 10
res = x % y
print(res)
# Output
# 5
# Here the Division operator divides x by y and returns the remainder
# The remainder is stored in the variable res
# The result 5 is then printed
Exponent Operator in Python (**)
- Exponent operator is denoted by a double asterisk '**' .
- Exponent operator or Exponentiation operator is also called power operator.
- In Exponent operator the left operand is raised to the power of right operand.
- Code Sample :
x = 4
y = 3
res = x ** y
print(res)
# Output
# 64
# Here the Exponent operator divides raises x by power y
# The result is stored in the variable res
# The result 64 is then printed
Prev. Tutorial : Types of operators
Next Tutorial : Assignment operators