Beginner Level
Intermediate Level
Advanced Level
Introduction
In Python, there are various ways to create and modify files, and appending data to an existing file is one of them. Appending to a file is a process of adding new content to the end of an existing file without losing the previous content. This tutorial will explain the different methods of appending to a file using Python. By the end of this tutorial, you will have a good understanding of the various methods of appending data to a file in Python programming language. Let's get started!
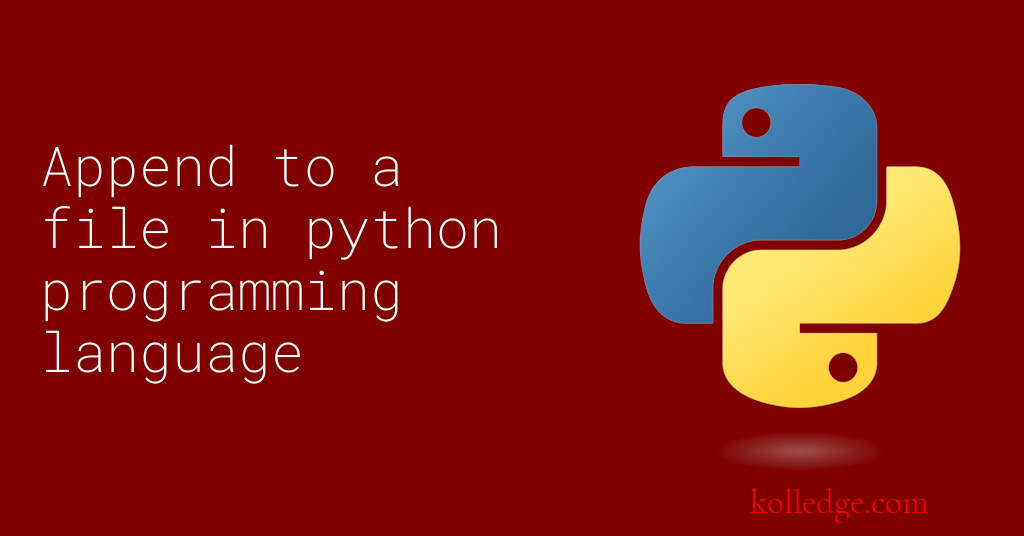
Table of Contents :
- How to append to a file in Python
- How to append and read on the Same File
- Writing to a Binary File
How to append to a file in Python :
- Open a file in append mode, using the
open()
method. - Write or append data to the file using the
write()
method. - Close the file using the
close()
method. - Code Sample :
file = open("example.txt", "a")
file.write("This line will be appended to the file.")
file.close()
How to append and read on the same File :
- Open a file in append-plus mode, using the
open()
method. - Write or append data to the file using the
write()
method. - Reset the file pointer to the beginning of the file using the
seek()
method with an argument of `0`, in order to read from the file. - Read the contents of the file using the
open()
method. - Close the file using the
close()
method. - Code Sample :
with open("example.txt", "a+") as file:
file.write("This line will be appended to the file.\n")
file.seek(0)
contents = file.read()
print(contents)
Writing to a Binary File
- Open a file in binary append mode, using the
open()
method. - Write or append binary data to the file using the
write()
method, with an argument of `b` preceding the binary data. - Close the file using the
close()
method. - Code Sample :
with open("example.bin", "ab") as file:
file.write(b"\x48\x65\x6c\x6c\x6f\x20\x57\x6f\x72\x6c\x64\x21")
# hexadecimal representation of "Hello World!"
Prev. Tutorial : Writing to a file
Next Tutorial : Check if a file exists