Beginner Level
Intermediate Level
Advanced Level
Introduction
String manipulation is a fundamental part of programming, and Python has a powerful set of tools to handle strings. In Python, a string is a sequence of characters in single or double quotes. Accessing individual characters within a string is a common operation that every programmer should know. In this tutorial, we will explore the different ways to access and manipulate the characters of a string in Python, as well as some common mistakes to avoid. So, let's get started and dive into the world of string manipulation in Python!
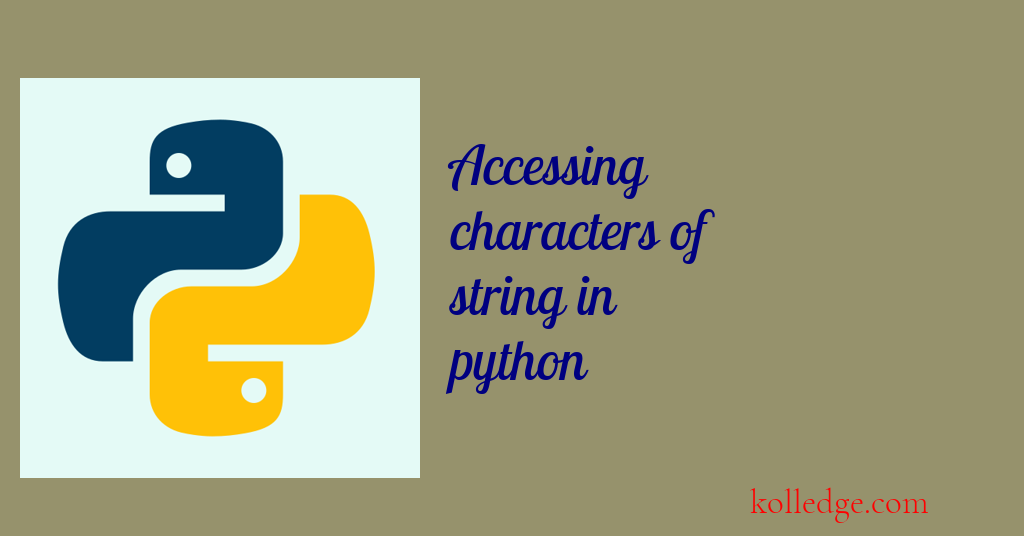
Table of Contents :
- Accessing characters of string
- Accessing a single character
- Accessing a range of characters
- Accessing every nth character
- Modifying characters of a string
Accessing characters of string :
- Different Ways of Accessing characters of string in python are :
- Accessing a single character
- Accessing a range of characters
- Accessing every nth character
Accessing a single character of a string:
- We can use the indexing operator
[ ]
with an index value to access a single character of a string. - Index values start from
0
for the first character and go up ton - 1
for a string of lengthn
. - We can use negative indexing to access characters from the end of the string with
-1
being the last character. - Example:
my_str = "Hello, world!"
print(my_str[0])
print(my_str[4])
print(my_str[-1])
print(my_str[-2])
# Output
# H
# o
# !
# d
Accessing a range of characters of a string:
- We can use the slicing operator
[ : ]
with start and end index values to access a range of characters of a string. - The range includes all characters from the start index up to but not including the end index.
- Example:
my_string = "Hello, world!"
print(my_string[0:5])
print(my_string[7:])
print(my_string[:5])
# Output
# Hello
# world!
# Hello
Accessing every nth character of a string:
- We can use the slicing operator
[start:end:step]
with a step value to access every nth character of a string. - Example:
my_string = "12345678"
print(my_string[::2])
# Output
# 1357
Modifying characters of a string:
- Unlike some other programming languages, Python strings are immutable,
- which means that once they are created, they cannot be modified.
- However, you can create a new string by slicing and concatenating parts of the original string.
- Example:
my_string = "Hello, world!"
new_string = my_string[:6] + "Kolledge!"
print(new_string)
# Output
# Hello,Kolledge!
Prev. Tutorial : Different String operations
Next Tutorial : Looping through a string